Vue Js Validate Aadhaar Number: To validate an Aadhaar number in Vue JS, you can use a regular expression to check if the input follows the Aadhaar number format. The Aadhaar number is a 12-digit number, so the regular expression should check if the input contains exactly 12 digits. To validate an Aadhaar number in Vue JS, you can use a regular expression (also known as RegEx) to check if the input follows the Aadhaar number format. A regular expression is a sequence of characters that defines a search pattern, which can be used to check if a string matches a specific format. In this case, we can use a regular expression to check if the input contains exactly 12 digits.
How to check Vue Js Aadhaar number is valid or not using Regular Expression
The given code snippet is a JavaScript function called formatAadhaarNumber()
. It performs the following tasks:
- It removes all non-digit characters from the input aadhaarNumber using the
replace()
function with a regular expression\D
, which matches any non-digit character. The resulting string is stored in the variableformattedInput
. - It adds a space after every four digits in the
formattedInput
string using thereplace()
function with another regular expression(\d{4})(\d)
and a replacement string'$1 $2'
. The regular expression matches four digits followed by a single digit, and the replacement string inserts a space between the fourth and fifth digit. - It repeats step 2 again to add another space after the next four digits.
- It sets the newly formatted input as the new value of the input field
aadhaarNumber
. - It defines a regular expression
aadhaarPattern
that matches a valid Aadhaar Number pattern. It checks whether the newly formatted input matches this pattern using thetest()
function. - If the input is empty, it sets the
errorMessage
to an empty string. - If the input matches the Aadhaar Number pattern, it sets the
errorMessage
to ‘Valid Aadhaar Number’. - If the input does not match the Aadhaar Number pattern, it sets the
errorMessage
to ‘Invalid Aadhaar Number’.
Overall, this function formats the input Aadhaar Number by adding spaces after every four digits and checks whether the formatted input matches a valid Aadhaar Number pattern.
Vue Js Validate Aadhaar Number Example
<div id="app">
<input type="text" v-model="aadhaarNumber" @input="formatAadhaarNumber" />
<pre v-if="errorMessage" style='color:red'>{{ errorMessage }}</pre>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
aadhaarNumber: '',
errorMessage: '',
}
},
methods: {
formatAadhaarNumber() {
// Remove all non-digit characters from the input
let formattedInput = this.aadhaarNumber.replace(/\D/g, '');
// Add a space after every four digits
formattedInput = formattedInput.replace(/(\d{4})(\d)/, '$1 $2');
formattedInput = formattedInput.replace(/(\d{4})\s(\d{4})(\d)/, '$1 $2 $3');
// Set the formatted input as the new value of the input field
this.aadhaarNumber = formattedInput;
const aadhaarPattern = /^[2-9]{1}[0-9]{3}\s[0-9]{4}\s[0-9]{4}$/;
if (this.aadhaarNumber === '') {
this.errorMessage = '';
} else if (aadhaarPattern.test(this.aadhaarNumber)) {
this.errorMessage = 'Valid Aadhaar Number';
} else {
this.errorMessage = 'Invalid Aadhaar Number';
}
},
},
});
app.mount('#app');
</script>
Output of Vue Js Validate Aadhaar Number
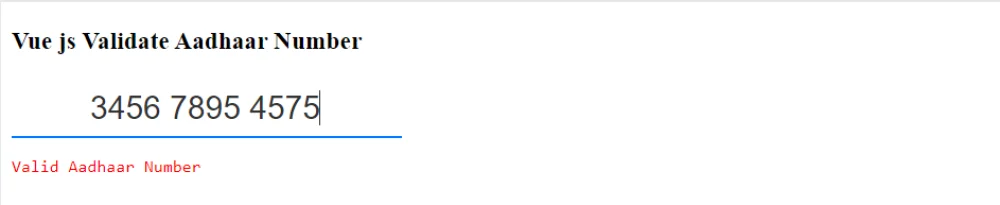